Web Driver
Introducing WebDriver The primary new feature in Selenium 2.0 is the integration of the WebDriver API. WebDriver is designed to provide a simpler, more concise.
Selenium WebDriver — Selenium Documentation
Abstract. This specification defines the WebDriver API, a platform and language-neutral interface that allows programs or scripts to introspect into, and control the.
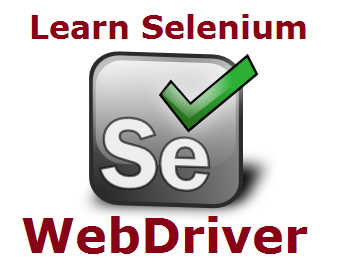
Selenium is a portable software testing framework for web applications. Selenium provides a record/playback tool for authoring tests without learning a test scripting.
Abstract. WebDriver is a remote control interface that enables introspection and control of user agents. It provides a platform- and language-neutral wire protocol as.
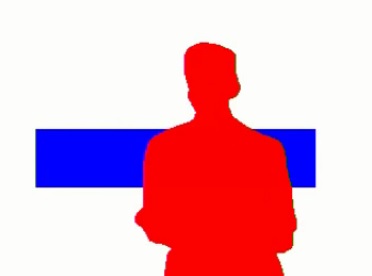
Welcome to the official website of The Web Drivers. The Web Drivers goes Global with a branch office in Tampa, Florida. We are celebrating by giving away the.
NOTE: We re currently working on documenting these sections.
We believe the information here is accurate, however be aware we are also still working on this
chapter. Additional information will be provided as we go which should make this chapter more
The primary new feature in Selenium 2.0 is the integration of the WebDriver API.
WebDriver is designed to provide a simpler, more concise programming interface in addition to
addressing some limitations in the Selenium-RC API. Selenium-WebDriver was developed
to better support dynamic web pages where elements of a page may change without the page
itself being reloaded. WebDriver s goal is to supply a well-designed object-oriented
API that provides improved support for modern advanced web-app testing problems.
How Does WebDriver Drive the Browser Compared to Selenium-RC.
Selenium-WebDriver makes direct calls to the browser using each browser s native support for automation.
How these direct calls are made, and the features they support depends on the browser you are using.
Information on each browser driver is provided later in this chapter.
For those familiar with Selenium-RC, this is quite different from what you are used to. Selenium-RC
worked the same way for each supported browser. It injected javascript functions into the browser
when the browser was loaded and then used its javascript to drive the AUT within the browser.
WebDriver does not use this technique. Again, it drives the browser directly using the browser s
built in support for automation.
WebDriver and the Selenium-Server
You may, or may not, need the Selenium Server, depending on how you intend to use Selenium-WebDriver.
If you will be only using the WebDriver API you do not need the Selenium-Server. If your browser
and tests will all run on the same machine, and your tests only use the WebDriver API, then you
do not need to run the Selenium-Server; WebDriver will run the browser directly.
There are some reasons though to use the Selenium-Server with Selenium-WebDriver.
You are using Selenium-Grid to distribute your tests over multiple machines or virtual machines VMs.
You want to connect to a remote machine that has a particular browser version that is not on
You are not using the Java bindings i.e. Python, C, or Ruby and would like to use HtmlUnit Driver
Setting Up a Selenium-WebDriver Project
To install Selenium means to set up a project in a development so you can write a program using
Selenium. How you do this depends on your programming language and your development environment.
The easiest way to set up a Selenium 2.0 Java project is to use Maven. Maven will download the
java bindings the Selenium 2.0 java client library and all its dependencies, and will create the
project for you, using a maven pom.xml project configuration file. Once you ve done this, you
can import the maven project into your preferred IDE, IntelliJ IDEA or Eclipse.
First, create a folder to contain your Selenium project files. Then, to use Maven, you need a
pom.xml file. This can be created with a text editor. We won t teach the
details of pom.xml files or for using Maven since there are already excellent references on this.
Your pom.xml file will look something like this. Create this file in the folder you created for
Be sure you specify the most current version. At the time of writing, the version listed above was
the most current, however there were frequent releases immediately after the release of Selenium 2.0.
Check the Maven download page for the current release and edit the above dependency accordingly.
Now, from a command-line, CD into the project directory and run maven as follows.
This will download Selenium and all its dependencies and will add them to the project.
Finally, import the project into your preferred development environment. For those not familiar
with this, we ve provided an appendix which shows this.
Importing a maven project into IntelliJ IDEA.
Importing a maven project into Eclipse.
As of Selenium 2.2.0, the C bindings are distributed as a set of signed dlls along with other
dependency dlls. Prior to 2.2.0, all Selenium dll s were unsigned.
To include Selenium in your project, simply download the latest
selenium-dotnet zip file from
If you are using Windows Vista or above, you should unblock the zip file before
unzipping it: Right click on the zip file, click Properties, click Unblock
Unzip the contents of the zip file, and add a reference to each of the unzipped
dlls to your project in Visual Studio or your IDE of choice.
If you are using Python for test automation then you probably are already familiar with developing
in Python. To add Selenium to your Python environment run the following command from
Pip requires pip to be installed, pip also has a dependency
Teaching Python development itself is beyond the scope of this document, however there are many
resources on Python and likely developers in your organization can help you get up to speed.
If you are using Ruby for test automation then you probably are already familiar with developing
in Ruby. To add Selenium to your Ruby environment run the following command from
gem install selenium-webdriver
Teaching Ruby development itself is beyond the scope of this document, however there are many
resources on Ruby and likely developers in your organization can help you get up to speed.
Perl bindings are provided by a third party, please refer to any of their documentation on how to
install / get started. There is one known Perl binding as of this writing.
For those who already have test suites written using Selenium 1.0, we have provided tips on how to
migrate your existing code to Selenium 2.0. Simon Stewart, the lead developer for Selenium 2.0,
has written an article on migrating from Selenium 1.0. We ve included this as an appendix.
Migrating From Selenium RC to Selenium WebDriver
Introducing the Selenium-WebDriver API by Example
WebDriver is a tool for automating web application testing, and in particular
to verify that they work as expected. It aims to provide a friendly API that s
easy to explore and understand, easier to use than the Selenium-RC 1.0 API,
which will help to make your tests easier to
read and maintain. It s not tied to any particular test framework, so it can
be used equally well in a unit testing or from a plain old main method.
This section introduces WebDriver s API and helps get you started becoming
familiar with it. Start by setting up a WebDriver project if you haven t already.
This was described in the previous section, Setting Up a Selenium-WebDriver Project.
Once your project is set up, you can see that WebDriver acts just as any normal library:
it is entirely self-contained, and you usually don t need to remember to start any
additional processes or run any installers before using it, as opposed to the proxy server
Note: additional steps are required to use ChromeDriver, Opera Driver, Android Driver
You re now ready to write some code. An easy way to get started is this
example, which searches for the term Cheese on Google and then outputs the
result page s title to the console.
package org.openqa.selenium.example;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.firefox.FirefoxDriver;
import org.openqa.selenium.support.ui.ExpectedCondition;
import org.openqa.selenium.support.ui.WebDriverWait;
public static void main String args
// Create a new instance of the Firefox driver
// Notice that the remainder of the code relies on the interface,
WebDriver driver new FirefoxDriver ;
// And now use this to visit Google
// Alternatively the same thing can be done like this
// Find the text input element by its name
WebElement element driver.findElement By.name q ;
// Enter something to search for
// Now submit the form. WebDriver will find the form for us from the element
// Check the title of the page
System.out.println Page title is: driver.getTitle ;
// Google s search is rendered dynamically with JavaScript.
// Wait for the page to load, timeout after 10 seconds
new WebDriverWait driver, 10. until new ExpectedConditionBoolean
public Boolean apply WebDriver d
return d.getTitle. toLowerCase. startsWith cheese. ;
// Should see: cheese. - Google Search
using OpenQA.Selenium.Firefox;
// Requires reference to WebDriver.Support.dll
using OpenQA.Selenium.Support.UI;
// Create a new instance of the Firefox driver.
// Further note that other drivers InternetExplorerDriver,
// ChromeDriver, etc. will require further configuration
// before this example will work. See the wiki pages for the
IWebDriver driver new FirefoxDriver ;
//Notice navigation is slightly different than the Java version
//This is because get is a keyword in C
IWebElement query driver.FindElement By.Name q ;
WebDriverWait wait new WebDriverWait driver, TimeSpan.FromSeconds 10 ;
wait.Until d return d.Title.ToLower. StartsWith cheese ; ;
// Should see: Cheese - Google Search
System.Console.WriteLine Page title is: driver.Title ;
from selenium import webdriver
from selenium.common.exceptions import TimeoutException
from selenium.webdriver.support.ui import WebDriverWait available since 2.4.0
from selenium.webdriver.support import expected_conditions as EC available since 2.26.0
Create a new instance of the Firefox driver
the page is ajaxy so the title is originally this:
find the element that s name attribute is q the google search box
inputElement driver.find_element_by_name q
inputElement.send_keys cheese.
submit the form although google automatically searches now without submitting
we have to wait for the page to refresh, the last thing that seems to be updated is the title
WebDriverWait driver, 10. until EC.title_contains cheese.
You should see cheese. - Google Search
driver Selenium::WebDriver.for :firefox
element driver.find_element :name q
puts Page title is driver.title
wait Selenium::WebDriver::Wait.new :timeout 10
wait.until driver.title.downcase.start_with. cheese.
puts Page title is driver.title
var driver new webdriver.Builder. build ;
var element driver.findElement webdriver.By.name q ;
driver.getTitle. then function title
console.log Page title is: title ;
return driver.getTitle. then function title
return title.toLowerCase. lastIndexOf cheese., 0 0;
Create a new instance of the driver
my driver Selenium::Remote::Driver- new;
Find the element that s name attribute is q google search box
my inputElement driver- find_element q, name ;
inputElement- send_keys cheese. ;
submit the form although google automatically searches now without submitting
Set the timeout for searching for elements to 10 seconds 0 by default
driver- set_implicit_wait_timeout 10000 ;
then use XPath to search for a page title containing cheese.
driver- find_element /html/head/title contains translate. , ABCDEFGHIJKLMNOPQRSTUVWXYZ, abcdefghijklmnopqrstuvwxyz, cheese. ;
In upcoming sections, you will learn more about how to use WebDriver for things
such as navigating forward and backward in your browser s history, and how to
test web sites that use frames and windows. We also provide a more
thorough discussions and examples.
Selenium-WebDriver API Commands and Operations
The first thing you re likely to want to do with WebDriver is navigate to a page.
The normal way to do this is by calling get :
Dependent on several factors, including the OS/Browser combination,
WebDriver may or may not wait for the page to load. In some circumstances,
WebDriver may return control before the page has finished, or even started, loading.
To ensure robustness, you need to wait for the element s to exist in the page using
Locating UI Elements WebElements
Locating elements in WebDriver can be done on the WebDriver instance itself or on a WebElement.
Each of the language bindings expose a Find Element and Find Elements method. The first returns
a WebElement object otherwise it throws an exception. The latter returns a list of WebElements, it can
return an empty list if no DOM elements match the query.
The Find methods take a locator or query object called By. By strategies are listed below.
This is the most efficient and preferred way to locate an element. Common pitfalls that UI developers
make is having non-unique id s on a page or auto-generating the id, both should be avoided. A class
on an html element is more appropriate than an auto-generated id.
Example of how to find an element that looks like this:
WebElement element driver.findElement By.id coolestWidgetEvah ;
IWebElement element driver.FindElement By.Id coolestWidgetEvah ;
element driver.find_element :id, coolestWidgetEvah
element driver.find_element_by_id coolestWidgetEvah
from selenium.webdriver.common.by import By
element driver.find_element by By.ID, value coolestWidgetEvah
element driver- find_element coolestWidgetEvah, id
Class in this case refers to the attribute on the DOM element. Often in practical use there are
many DOM elements with the same class name, thus finding multiple elements becomes the more practical
option over finding the first element.
class cheese Cheddar class cheese Gouda
ListWebElement cheeses driver.findElements By.className cheese ;
IListIWebElement cheeses driver.FindElements By.ClassName cheese ;
cheeses driver.find_elements :class_name, cheese
cheeses driver.find_elements :class, cheese
cheeses driver.find_elements_by_class_name cheese
cheeses driver.find_elements By.CLASS_NAME, cheese
cheeses driver- find_elements cheese, class ;
The DOM Tag Name of the element.
WebElement frame driver.findElement By.tagName iframe ;
IWebElement frame driver.FindElement By.TagName iframe ;
frame driver.find_element :tag_name, iframe
frame driver.find_element_by_tag_name iframe
frame driver.find_element By.TAG_NAME, iframe
frame driver- find_element iframe, tag_name ;
Find the input element with matching name attribute.
WebElement cheese driver.findElement By.name cheese ;
IWebElement cheese driver.FindElement By.Name cheese ;
cheese driver.find_element :name, cheese
cheese driver.find_element_by_name cheese
cheese driver.find_element By.NAME, cheese
cheese driver- find_element cheese, name ;
Find the link element with matching visible text.
WebElement cheese driver.findElement By.linkText cheese ;
IWebElement cheese driver.FindElement By.LinkText cheese ;
cheese driver.find_element :link_text, cheese
cheese driver.find_element :link, cheese
cheese driver.find_element_by_link_text cheese
cheese driver.find_element By.LINK_TEXT, cheese
cheese driver- find_element cheese, link_text ;
Find the link element with partial matching visible text.
WebElement cheese driver.findElement By.partialLinkText cheese ;
IWebElement cheese driver.FindElement By.PartialLinkText cheese ;
cheese driver.find_element :partial_link_text, cheese
cheese driver.find_element_by_partial_link_text cheese
cheese driver.find_element By.PARTIAL_LINK_TEXT, cheese
cheese driver- find_element cheese, partial_link_text ;
Like the name implies it is a locator strategy by css. Native browser support
is used by default, so please refer to w3c css selectors for a list of generally available css selectors. If a browser does not have
native support for css queries, then Sizzle is used. IE 6,7 and FF3.0
currently use Sizzle as the css query engine.
Beware that not all browsers were created equal, some css that might work in one version may not work
Example of to find the cheese below:
id food class dairy milk class dairy aged cheese
WebElement cheese driver.findElement By.cssSelector food span.dairy.aged ;
IWebElement cheese driver.FindElement By.CssSelector food span.dairy.aged ;
cheese driver.find_element :css, food span.dairy.aged
cheese driver.find_element_by_css_selector food span.dairy.aged
cheese driver.find_element By.CSS_SELECTOR, food span.dairy.aged
cheese driver- find_element food span.dairy.aged, css ;
At a high level, WebDriver uses a browser s native XPath capabilities wherever
possible. On those browsers that don t have native XPath support, we have
provided our own implementation. This can lead to some unexpected behaviour
unless you are aware of the differences in the various xpath engines.
This is a little abstract, so for the following piece of HTML:
ListWebElement inputs driver.findElements By.xpath //input ;
IListIWebElement inputs driver.FindElements By.XPath //input ;
inputs driver.find_elements :xpath, //input
inputs driver.find_elements_by_xpath //input
inputs driver.find_elements By.XPATH, //input
inputs driver- find_elements //input
The following number of matches will be found
Sometimes HTML elements do not need attributes to be explicitly declared
because they will default to known values. For example, the input tag does
not require the type attribute because it defaults to text. The rule of
thumb when using xpath in WebDriver is that you should not expect to be able
to match against these implicit attributes.
You can execute arbitrary javascript to find an element and as long as you return a DOM Element,
it will be automatically converted to a WebElement object.
Simple example on a page that has jQuery loaded:
WebElement element WebElement JavascriptExecutor driver. executeScript return . cheese 0 ;
IWebElement element IWebElement IJavaScriptExecutor driver. ExecuteScript return . cheese 0 ;
element driver.execute_script return . cheese 0
element driver- execute_script return . cheese 0 ;
Finding all the input elements to the every label on a page:
ListWebElement labels driver.findElements By.tagName label ;
ListWebElement inputs ListWebElement JavascriptExecutor driver. executeScript
var labels arguments 0, inputs ; for var i 0; i
inputs.push document.getElementById labels i. getAttribute for ; return inputs;, labels ;
IListIWebElement labels driver.FindElements By.TagName label ;
IListIWebElement inputs IListIWebElement IJavaScriptExecutor driver. ExecuteScript
labels driver.find_elements :tag_name, label
inputs.push document.getElementById labels i. getAttribute for ; return inputs;, labels
labels driver.find_elements_by_tag_name label
my labels driver- find_elements label, tag_name ;
my inputs driver- execute_script var labels arguments, inputs ; for var i 0; i, labels ;
Moving Between Windows and Frames
Some web applications have many frames or multiple windows. WebDriver supports
moving between named windows using the switchTo method:
driver.switchTo. window windowName ;
driver.switch_to.window windowName
driver- switch_to_window windowName ;
All calls to driver will now be interpreted as being directed to the
particular window. But how do you know the window s name. Take a look at the
javascript or link that opened it:
href somewhere.html target windowName Click here to open a new window
Alternatively, you can pass a window handle to the switchTo. window
method. Knowing this, it s possible to iterate over every open window like so:
for String handle : driver.getWindowHandles
driver.switchTo. window handle ;
driver.window_handles.each do handle
driver.switch_to.window handle
for handle in driver.window_handles:
windows driver- get_window_handles
driver- switch_to_window window ;
You can also switch from frame to frame or into iframes :
driver.switchTo. frame frameName ;
driver.switch_to.frame frameName
driver- switch_to_frame frameName ;
Navigation: History and Location
Earlier, we covered navigating to a page using the get command
driver.get As you ve seen, WebDriver has a
number of smaller, task-focused interfaces, and navigation is a useful task.
Because loading a page is such a fundamental requirement, the method to do this
lives on the main WebDriver interface, but it s simply a synonym to:
driver.get python doesn t have driver.navigate
To reiterate: navigate. to and get do exactly the same thing.
One s just a lot easier to type than the other.
The navigate interface also exposes the ability to move backwards and forwards in your browser s history:
Please be aware that this functionality depends entirely on the underlying
browser. It s just possible that something unexpected may happen when you call
these methods if you re used to the behaviour of one browser over another.
Before we leave these next steps, you may be interested in understanding how to
use cookies. First of all, you need to be on the domain that the cookie will be
valid for. If you are trying to preset cookies before
you start interacting with a site and your homepage is large / takes a while to load
an alternative is to find a smaller page on the site, typically the 404 page is small
// Now set the cookie. This one s valid for the entire domain
Cookie cookie new Cookie key, value ;
driver.manage. addCookie cookie ;
// And now output all the available cookies for the current URL
SetCookie allCookies driver.manage. getCookies ;
for Cookie loadedCookie : allCookies
System.out.println String.format s - s, loadedCookie.getName, loadedCookie.getValue ;
// You can delete cookies in 3 ways
driver.manage. deleteCookieNamed CookieName ;
driver.manage. deleteCookie loadedCookie ;
driver.manage. deleteAllCookies ;
Now set the cookie. Here s one for the entire domain
the cookie name here is key and its value is value
driver.add_cookie name : key, value : value, path : /
additional keys that can be passed in are:
expiry - Milliseconds since the Epoch it should expire.
And now output all the available cookies for the current URL
for cookie in driver.get_cookies :
print s - s cookie name, cookie value
You can delete cookies in 2 ways
driver.delete_cookie CookieName
driver.manage.add_cookie :name key, :value value
:path String, :secure - Boolean, :expires - Time, DateTime, or seconds since epoch
driver.manage.all_cookies.each cookie
puts cookie :name cookie :value
driver.manage.delete_cookie CookieName
driver.manage.delete_all_cookies
the cookie name here is key and its value is value
driver- add_cookie key, value, /, example.com, 0 ;
additional required inputs are path and domain
the final input secure is an optional boolean
my cookies_ref driver- get_all_cookies ; Returns reference to AoH
printf s s n, cookie_ref- name, cookie_ref- value ;
driver- delete_cookie_named key ;
This is easy with the Firefox Driver:
FirefoxProfile profile new FirefoxProfile ;
profile.addAdditionalPreference general.useragent.override, some UA string ;
WebDriver driver new FirefoxDriver profile ;
profile Selenium::WebDriver::Firefox::Profile.new
profile general.useragent.override some UA string
driver Selenium::WebDriver.for :firefox, :profile profile
profile webdriver.FirefoxProfile
profile.set_preference general.useragent.override, some UA string
driver webdriver.Firefox profile
use Selenium::Remote::Driver::Firefox::Profile;
my profile Selenium::Remote::Driver::Firefox::Profile- new;
profile- set_preference general.useragent.overide some UA string ;
my driver Selenium::Remote::Driver- new firefox_profile profile ;
Here s an example of using the Actions class to perform a drag and drop.
Native events are required to be enabled.
WebElement element driver.findElement By.name source ;
WebElement target driver.findElement By.name target ;
new Actions driver. dragAndDrop element, target. perform ;
element driver.find_element :name source
target driver.find_element :name target
driver.action.drag_and_drop element, target. perform
from selenium.webdriver.common.action_chains import ActionChains
element driver.find_element_by_name source
target driver.find_element_by_name target
ActionChains driver. drag_and_drop element, target. perform
Driver Specifics and Tradeoffs
WebDriver is the name of the key interface against which tests should be
written, but there are several implementations. These include:
This is currently the fastest and most lightweight implementation of WebDriver.
As the name suggests, this is based on HtmlUnit. HtmlUnit is a java based implementation
of a WebBrowser without a GUI. For any language binding other than java the
Selenium Server is required to use this driver.
WebDriver driver new HtmlUnitDriver ;
IWebDriver driver new RemoteWebDriver new Uri
DesiredCapabilities.HtmlUnit ;
driver webdriver.Remote webdriver.DesiredCapabilities.HTMLUNIT.copy
driver Selenium::WebDriver.for :remote, :url :desired_capabilities :htmlunit
my driver Selenium::Remote::Driver- new browser_name htmlunit, remote_server_addr localhost, port 4444 ;
Fastest implementation of WebDriver
A pure Java solution and so it is platform independent.
Emulates other browsers JavaScript behaviour see below
JavaScript in the HtmlUnit Driver
None of the popular browsers uses the JavaScript engine used by HtmlUnit
Rhino. If you test JavaScript using HtmlUnit the results may differ
significantly from those browsers.
When we say JavaScript we actually mean JavaScript and the DOM. Although
the DOM is defined by the W3C each browser has its own quirks and differences
in their implementation of the DOM and in how JavaScript interacts with it.
HtmlUnit has an impressively complete implementation of the DOM and has good
support for using JavaScript, but it is no different from any other
browser: it has its own quirks and differences from both the W3C standard and
the DOM implementations of the major browsers, despite its ability to mimic
With WebDriver, we had to make a choice; do we enable HtmlUnit s JavaScript
capabilities and run the risk of teams running into problems that only manifest
themselves there, or do we leave JavaScript disabled, knowing that there are
more and more sites that rely on JavaScript. We took the conservative approach,
and by default have disabled support when we use HtmlUnit. With each release of
both WebDriver and HtmlUnit, we reassess this decision: we hope to enable
JavaScript by default on the HtmlUnit at some point.
If you can t wait, enabling JavaScript support is very easy:
HtmlUnitDriver driver new HtmlUnitDriver true ;
WebDriver driver new RemoteWebDriver new Uri
DesiredCapabilities.HtmlUnitWithJavaScript ;
caps Selenium::WebDriver::Remote::Capabilities.htmlunit :javascript_enabled true
driver Selenium::WebDriver.for :remote, :url :desired_capabilities caps
driver webdriver.Remote webdriver.DesiredCapabilities.HTMLUNITWITHJS
driver new Selenium::Remote::Driver browser_name firefox, port 4444, version, platform LINUX, javascript 1, auto_close 1 ;
This will cause the HtmlUnit Driver to emulate Firefox 3.6 s JavaScript
Controls the Firefox browser using a Firefox plugin.
The Firefox Profile that is used is stripped down from what is installed on the
machine to only include the Selenium WebDriver.xpi plugin. A few settings are
also changed by default see the source to see which ones
Firefox Driver is capable of being run and is tested on Windows, Mac, Linux.
Currently on versions 3.6, 10, latest - 1, latest
Slower than the HtmlUnit Driver
Suppose that you wanted to modify the user agent string as above, but you ve
got a tricked out Firefox profile that contains dozens of useful extensions.
There are two ways to obtain this profile. Assuming that the profile has been
created using Firefox s profile manager firefox -ProfileManager :
ProfilesIni allProfiles new ProfilesIni ;
FirefoxProfile profile allProfiles.getProfile WebDriver ;
profile.setPreferences foo.bar, 23 ;
Alternatively, if the profile isn t already registered with Firefox:
File profileDir new File path/to/top/level/of/profile ;
FirefoxProfile profile new FirefoxProfile profileDir ;
profile.addAdditionalPreferences extraPrefs ;
As we develop features in the Firefox Driver, we expose the ability to use them.
For example, until we feel native events are stable on Firefox for Linux, they
are disabled by default. To enable them:
profile.setEnableNativeEvents true ;
profile.native_events_enabled True
The InternetExplorerDriver is a standalone server which implements WebDriver s wire protocol. This driver has been tested with IE 7, 8, 9, 10, and 11 on appropriate combinations of Vista, Windows 7, Windows 8, and Windows 8.1. As of 15 April 2014, IE 6 is no longer supported.
The driver supports running 32-bit and 64-bit versions of the browser. The choice of how to determine which bit-ness to use in launching the browser depends on which version of the IEDriverServer.exe is launched. If the 32-bit version of IEDriverServer.exe is launched, the 32-bit version of IE will be launched. Similarly, if the 64-bit version of IEDriverServer.exe is launched, the 64-bit version of IE will be launched.
WebDriver driver new InternetExplorerDriver ;
IWebDriver driver new InternetExlorerDriver ;
driver Selenium::WebDriver.for :ie
my driver Selenium::Remote::Driver- new browser_name internet explorer ;
Runs in a real browser and supports Javascript
Obviously the InternetExplorerDriver will only work on Windows.
Comparatively slow though still pretty snappy.
ChromeDriver is maintained / supported by the Chromium
project iteslf. WebDriver works with Chrome through the chromedriver binary found on the chromium
project s download page. You need to have both chromedriver and a version of chrome browser installed.
chromedriver needs to be placed somewhere on your system s path in order for WebDriver to automatically
discover it. The Chrome browser itself is discovered by chromedriver in the default installation path.
These both can be overridden by environment variables. Please refer to the wiki
WebDriver driver new ChromeDriver ;
IWebDriver driver new ChromeDriver ;
driver Selenium::WebDriver.for :chrome
my driver Selenium::Remote::Driver- new browser_name chrome ;
Runs in a real browser and supports JavaScript
Because Chrome is a Webkit-based browser, the ChromeDriver may allow you to
verify that your site works in Safari. Note that since Chrome uses its own V8
JavaScript engine rather than Safari s Nitro engine, JavaScript execution may
See either the ios-driver or appium projects.
Alternative Back-Ends: Mixing WebDriver and RC Technologies
The Java version of WebDriver provides an implementation of the Selenium-RC API. These means that
you can use the underlying WebDriver technology using the Selenium-RC API. This is primarily
provided for backwards compatibility. It allows those who have existing test suites using the
Selenium-RC API to use WebDriver under the covers. It s provided to help ease the migration path
to Selenium-WebDriver. Also, this allows one to use both APIs, side-by-side, in the same test code.
Selenium-WebDriver is used like this:
// You may use any WebDriver implementation. Firefox is used here as an example
// A base url, used by selenium to resolve relative URLs
// Create the Selenium implementation
Selenium selenium new WebDriverBackedSelenium driver, baseUrl ;
// Perform actions with selenium
selenium.type name q, cheese ;
// Get the underlying WebDriver implementation back. This will refer to the
// same WebDriver instance as the driver variable above.
WebDriver driverInstance WebDriverBackedSelenium selenium. getWrappedDriver ;
//Finally, close the browser. Call stop on the WebDriverBackedSelenium instance
//instead of calling driver.quit. Otherwise, the JVM will continue running after
//the browser has been closed.
Allows for the WebDriver and Selenium APIs to live side-by-side
Provides a simple mechanism for a managed migration from the Selenium RC API
Does not require the standalone Selenium RC server to be run
Does not implement every method
More advanced Selenium usage using browserbot or other built-in JavaScript
methods from Selenium Core may not work
Some methods may be slower due to underlying implementation differences
Backing WebDriver with Selenium
WebDriver doesn t support as many browsers as Selenium RC does, so in order to
provide that support while still using the WebDriver API, you can make use of
Safari is supported in this way with the following code be sure to disable
DesiredCapabilities capabilities new DesiredCapabilities ;
capabilities.setBrowserName safari ;
CommandExecutor executor new SeleneseCommandExecutor new URL new URL capabilities ;
WebDriver driver new RemoteWebDriver executor, capabilities ;
There are currently some major limitations with this approach, notably that
findElements doesn t work as expected. Also, because we re using Selenium Core
for the heavy lifting of driving the browser, you are limited by the JavaScript
Running Standalone Selenium Server for use with RemoteDrivers
From Selenium s Download page download selenium-server-standalone-.jar and optionally IEDriverServer. If you plan to work with Chrome, download it from Google Code.
Unpack IEDriverServer and/or chromedriver and put them in a directory which is on the PATH / PATH - the Selenium Server should then be able to handle requests for IE / Chrome without additional modifications.
Start the server on the command line with
java -jar /selenium-server-standalone-.jar
If you want to use native events functionality, indicate this on the command line with the option
-Dwebdriver.enable.native.events 1
For other command line options, execute
java -jar /selenium-server-standalone-.jar -help
In order to function properly, the following ports should be allowed incoming TCP connections: 4444, 7054-5 or twice as many ports as the number of concurrent instances you plan to run. Under Windows, you may need to unblock the applications as well.
You can find further resources for WebDriver
Of course, don t hesitate to do an internet search on any Selenium topic, including
Selenium-WebDriver s drivers. There are quite a few blogs on Selenium along with numerous posts
on various user forums. Additionally the Selenium User s Group is a great resource.
This chapter has simply been a high level walkthrough of WebDriver and some of its key
capabilities. Once getting familiar with the Selenium-WebDriver API you will then want to learn
how to build test suites for maintainability, extensibility, and reduced fragility when features of
the AUT frequently change. The approach most Selenium experts are now recommending is to design
your test code using the Page Object Design Pattern along with possibly a Page Factory.
Selenium-WebDriver provides support for this by supplying a PageFactory class in Java and C.
This is presented, along with other advanced topics, in the
next chapter. Also, for high-level description of this
technique, you may want to look at the
Test Design Considerations chapter. Both of these
chapters present techniques for writing more maintainable tests by making your test code more
WebDriver is a clean, fast framework for automated testing of webapps. Why is it needed. And what problems does it solve that existing frameworks don t address.
.jpg)
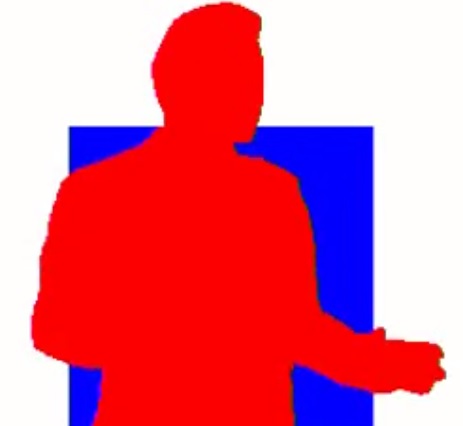
Selenium WebDriver. The biggest change in Selenium recently has been the inclusion of the WebDriver API. Driving a browser natively as a user would either locally or.
Feb 08, 2014 The 5 Minute Getting Started Guide. WebDriver is a tool for automating testing web applications, and in particular to verify that they work as expected.